Google offers Firebase Realtime Database, a cloud-hosted NoSQL database, as a component of the Firebase product suite. It offers programmers a scalable and adaptable way to create real-time applications that need data to be synchronized across several clients.
Features of Firebase Realtime Database
Real-time Data Sync
You may synchronize data in real-time between various clients, including web, mobile, and backend servers, with the help of Firebase Realtime Database. All connected clients receive instantaneous updates to any changes made to the database, guaranteeing accurate and consistent data.
NoSQL Database Structure
The Realtime Database stores data in a versatile format akin to JSON using a NoSQL data paradigm. This facilitates the storing and retrieval of complicated data by enabling dynamic and hierarchical data structures.
Offline Capabilities
The Firebase Realtime Database has offline support by default. Data is automatically cached locally by the database when a device loses connectivity. Data consistency is ensured when the device reconnects and the local modifications are synchronized with the server.
Integration with Firebase Services
Cloud Functions, Cloud Messaging, and Firebase Authentication are just a few of the Firebase services that the Firebase Realtime Database easily connects with. You may create robust and all-inclusive serverless functionalities, push notifications, and user management solutions with this integration.
SDKs and Libraries
Flutter, iOS, Android, and web are just a few of the platforms for which Firebase Realtime Database provides SDKs. These SDKs make it easy to incorporate the database into your apps by offering a straightforward and standardized API for doing so.
Security and Authentication
You can manage who has access to your database with Firebase’s strong security rules. To make sure that only authorized users can view and write data, you can design rules based on authentication, user roles, and data validation.
Performance and Scalability
Firebase Realtime Database adapts automatically to the demands of your application. Applications requiring real-time response and enormous traffic volumes can benefit from its low latency data access and ability to manage millions of concurrent connections.
1. Prerequisites
Before we get started, make sure you have the following installed:
– Firebase account
– Firebase SDK for Flutter
– Physical device(iOS/Android) / Emulator
– Android Studio / Other Editor
– Basic Flutter Knowledge
2. Setting up Firebase Project
- Go to Firebase Console
- Click on ‘Add Project’ and complete all steps one by one.
- For manual set up follow Link
- For Automatic set up follow Link
Note : don’t forgot add google-service.json for android and GoogleService-info.plist File to their respective folder.
3. Add Firebase Dependency
- Now let’s focus on code
- First of all you need to add below dependency in pubspec.yaml
firebase_core: ^2.32.0
firebase_database: ^10.5.7
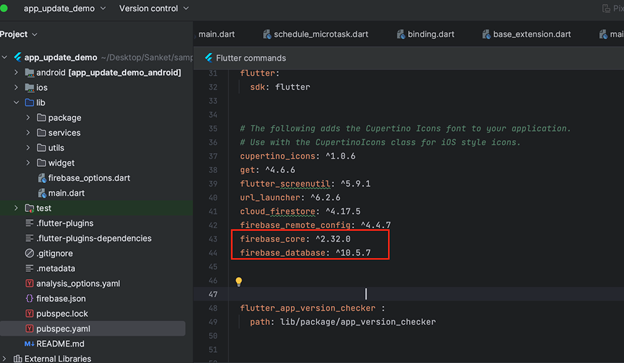
Apply command – – flutter clean & flutter pub get
4. Initialize Firebase
- Add below code in main() method before RunApp();
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp(options:DefaultFirebaseOptions.currentPlatform);
like the below example
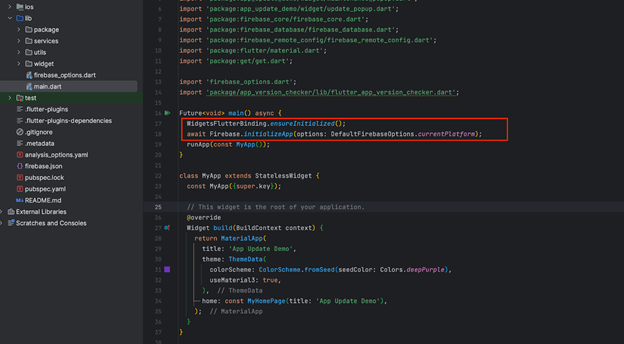
5. Firebase Active Realtime Database
- Firebase console>All Product > Realtime Database
- Create Database
- Go to Realtime Database > Rules
- Set Rules like this
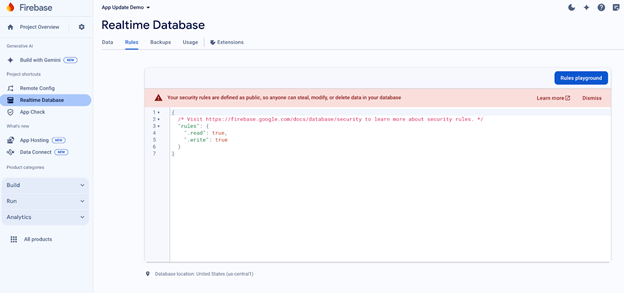
6. Let’s Create User
Create Method
// Add data to firebase
addUserData() { DatabaseReference databaseReference = FirebaseDatabase.instance.ref().child('users'); databaseReference.push().set({ 'name': txtName.text.trim(), 'email': txtEmail.text.trim(), }).then((value) => {txtName.clear(), txtEmail.clear()});
}
- Add name and email in firebase realtime db
- Then fetch data
Create instant of db
DatabaseReference databaseReference = FirebaseDatabase.instance.ref().child('users');
Now create listener like example below
databaseReference.onValue.listen((event) { DataSnapshot dataSnapshot = event.snapshot; if (dataSnapshot.exists) { Map<dynamic, dynamic>? values = dataSnapshot.value as Map; values.forEach((key, values) { Map<String, dynamic> typedValues = values.cast<String, dynamic>(); userList.add(UserModel.fromMap(typedValues)); debugPrint('userList --------> ${userList.length}'); }); }
});
Whenever data is added and changed listener will give a new event and manage data as per need.
Example
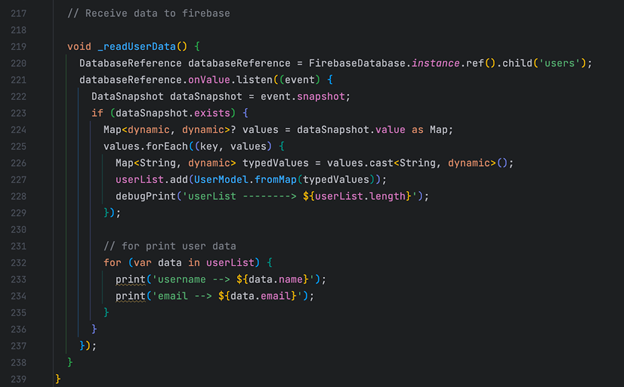
Here is my user model
class UserModel { UserModel({ this.name, this.email, }); final String? name; final String? email; Map<String, dynamic> toMap() { return <String, dynamic>{ 'name': name, 'email': email, }; } factory UserModel.fromMap(Map<String, dynamic> map) { return UserModel( name: map['name'] as String, email: map['email'] as String, ); }
}
Final Result
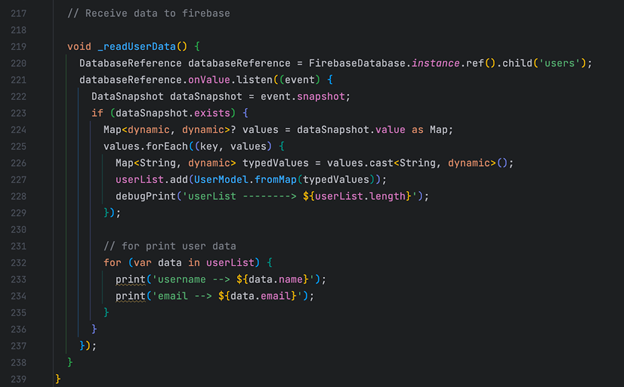
Conclusion
We looked at the strong features of Firebase Realtime Database and how it works with Flutter to allow real-time data management in your mobile apps in this extensive guide. In your Flutter project, we began by configuring Firebase. We then guided you through the process of integrating the Firebase Realtime Database into your application.
Now that you have a firm grasp on the characteristics of Firebase Realtime Database and how to use them in Flutter, you can advance the creation of mobile apps.
To create captivating and responsive apps that offer a smooth and dynamic user experience, start utilizing the power of Firebase Realtime Database and hire Flutter app developers now.